#Quotation web services (QuotationV2Ws)
Provides service methods to handle quotation operations.
* **Type**: SOAP
* **Production WSDL**: https://services.generali.gr/soap/v2/quotation?wsdl
* **Test WSDL**: https://services-test.generali.gr/soap/v2/quotation?wsdl
Methods
* [tariffy](#tariffy)
* [issue](#issue)
* [listPackagesInfo](#listPackagesInfo)
* [getPackageInfo](#getPackageInfo)
* [listOpenPendencies](#listOpenPendencies)
* [uploadPendency](#uploadPendency)
* [getQuotationDocument](#getQuotationDocument)
* [getQuotationStatus](#getQuotationStatus)
##tariffy
Tariffies the selected coverages of an insurable object. Notice that regarding the covers, only the *missing* information is required. This includes the sum insured, the parameters, and rarely the premium of the covers that are not disabled as well as those covers that are optional (even if they contain no extra input information). Any other data sent will be ignored by the tariffication engine.
In order to know which values should be sent in your request you could:
* Use our [listPackagesInfo](#listPackagesInfo) *(prefered)* or the [getPackageInfo](#getPackageInfo) : Check the list of mandatory and optional covers in order to know which ones can be selected. Check the [cover field](#FieldInfo) type values to know which of them (if any) should be sent with their cover.
* Use OneView as a reference: Check the list of mandatory and optional covers in order to know which ones can be selected. Check the inputs that are capable of taking values from the user (they are not disabled), those are the ones that should be sent along with their cover.
Check the examples to get a better idea of how to use the service.
###Input
Field | Type | Value | Required/Optional | Description | Example
----- | ---- | ----- | ----------------- | ----------- | -------
distChannelCode | String (1-5 chars) | Numerical code | Required | The 5 digit code representing the distribution channel | 50072
duration | Integer | **1**: Annual **2**: Semiannual **4**: Terminally | Required | The duration of the policy | 1
insuranceStartDate | Date | Date | Required | The start date of the policy's insurance period | 2015-11-23
policyHolder | Person | Person | Optional if insurable contains a Person already | The policy holder | *(see below)*
insurable | Insurable | MotorInsurable, so far | Required | The object insured by the policy | *(see below)*
pack | Pack | Pack | Required | The package to tariffy (including extra products and parameters) | *(see below)*
####Pack
Field | Type | Value | Required/Optional | Description | Example
----- | ---- | ----- | ----------------- | ----------- | -------
code | String | Numerical | Required | The code of the package | 123000000
products | List[Cover] | Cover | Required | The chosen products of the package | List["123000000", Value[20223.23], Value["100"], List["paramType", "400", Value[1000.50]]]
####Cover
Field | Type | Value | Required/Optional | Description | Example
----- | ---- | ----- | |---------------- | ----------- | -------
code | String | String | Required | The code of the product | 234
sumInsured | Value | Value | Optional | The sum insured of the product | Value[20223.23]
premium | Value | Value | Otional | The premium of the product | Value["100"]
params | List[CoverParam] | CoverParam | Optional | The params of the product | List["paramType", "400", Value[1000.50]]
####CoverParam
Field | Type | Value | Required/Optional | Description | Example
----- | ---- | ----- | ----------------- | ----------- | -------
type | String | String | Required | The type of the product param | "GGY"
code | String | String | Required | The code of the product param | "400"
####Value
Can be choosen either the codeValue or the numericalValue.
Field | Type | Value | Required/Optional | Description | Example
----- | ---- | ----- | |---------------- | ----------- | -------
codeValue | String | String | Required | The value as code | 200
numericalValue | BigDecimal | BigDecimal | Required | The value as number | 5000.43
###Output
Field | Type | Value | Description | Example
----- | ---- | ----- | ----------- | -------
requestCode | String | String | Is the key code of the tariffication in case of tracking it down | 162
totalPremiumGross | BigDecimal | BigDecimal | Tariffication's total premium gross amount | 450.67
totalPremiumNet | BigDecimal | BigDecimal | Tariffication's total premium net amount | 390.81
coverageList | Package | Package | The selected coverages and products | *(see above)*
###Example 1
In this example, taken from OneView, we tariffy package Speed 2 with different policy holder from driver and get a valid result. Notice that only the *missing* information is sent (red circles), which is the one coming from the the non disabled inputs.
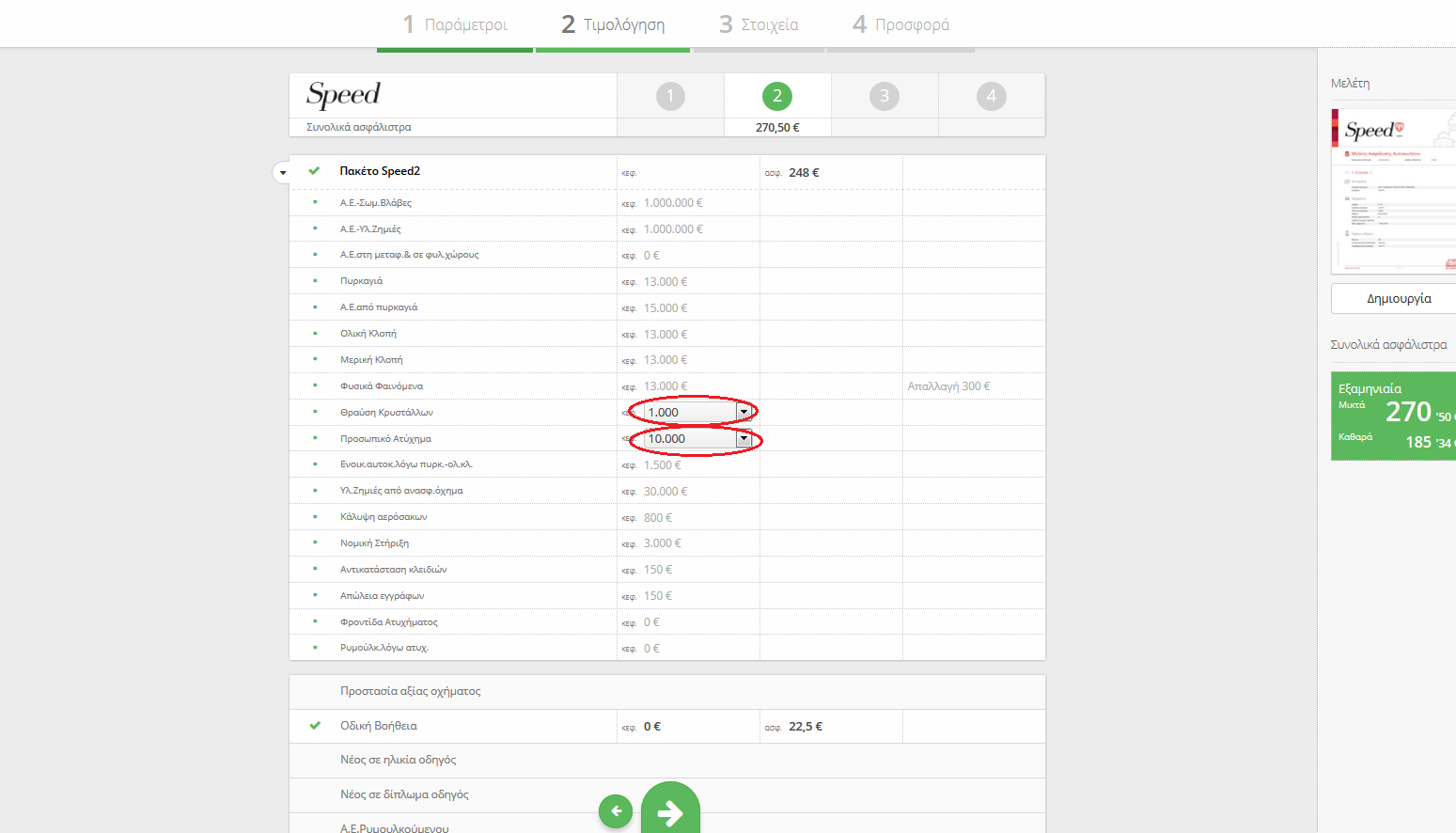
*Input*
```
0800012015-11-2412345678911962-08-22000201012940356032IBZ8972101982-08-221500 011genderCode>
953950
```
*Output*
```
req-08000-1493206558206244.27356.831000000.001000000.000.0012940.0015000.0012940.0012940.0012940.009501000.0095310000.001500.0030000.00800.003000.00150.00150.000.000.00
```
###Example 2
In this example, taken from OneView, we tariffy package Speed 2 with the same policy holder and driver and get a valid result.
*Input*
```
0800012015-11-24000201012940356032IBZ8972101982-08-221500 011genderCode>
953950
```
*Output*
```
req-08000-1493206649927244.27356.831000000.001000000.000.0012940.0015000.0012940.0012940.0012940.009501000.0095310000.001500.0030000.00800.003000.00150.00150.000.000.00
```
#### Client example (in Java)
Example of a simple Java client. Notice that the name of the classes depends entirely on your client generator and it may vary from the following.
```
import java.math.BigDecimal;
import java.util.GregorianCalendar;
import java.util.List;
import java.util.Map;
import java.util.logging.Level;
import java.util.logging.Logger;
import javax.xml.datatype.DatatypeConfigurationException;
import javax.xml.datatype.DatatypeFactory;
import javax.xml.ws.BindingProvider;
public class TarifficationExample1 {
public static final void main(String[] args) {
Soap_002fV2_002fQuotation service = new Soap_002fV2_002fQuotation();
QuotationWsV2 quotationService = service.getQuotationWsV2BasePort();
Map context = ((BindingProvider)quotationService).getRequestContext();
context.put(BindingProvider.USERNAME_PROPERTY, "ws-000000028ezr9");
context.put(BindingProvider.PASSWORD_PROPERTY, "CP64UpfDVZ-test");
Person driver = new Person();
driver.setBirthDate(date(1982, 8, 22));
driver.setMaritalStatusCode(1);
driver.setGenderCode(1);
driver.setPostalCode("500 01");
MotorInsurable motorInsurable = new MotorInsurable();
motorInsurable.setMotorUseCode("000");
motorInsurable.setEurotaxCode("35603");
motorInsurable.setManufacturerYear(2010);
motorInsurable.setMarketValue(BigDecimal.valueOf(15650));
motorInsurable.setPlateNo("IBZ8976");
motorInsurable.setNoOfClaims(2);
motorInsurable.setDriver(driver);
// Fill mandatory Cover for Personal Accident
Cover personalAccident = new Cover();
Value sumInsuredPersonal = new Value();
sumInsuredPersonal.setCodeValue("953");
personalAccident.setCode("280000000");
personalAccident.setSumInsured(sumInsuredPersonal);
// Fill mandatory Cover for Windscreen
Cover windscreen = new Cover();
Value sumInsuredWindscreen = new Value();
sumInsuredWindscreen.setCodeValue("950");
windscreen.setCode("281000000");
windscreen.setSumInsured(sumInsuredWindscreen);
// Fill optional Cover for Motor Assistance
Cover motorAssistance = new Cover();
motorAssistance.setCode("753450171");
Pack pack = new Pack();
pack.setCode("802");
pack.getCover().add(personalAccident);
pack.getCover().add(windscreen);
pack.getCover().add(motorAssistance);
String distChannelCode = "08000";
int duration = 1;
Person policyHolder = null;
try {
Tariffication tariffication = quotationService.tariffy(distChannelCode, duration, date(2015, 11, 23), policyHolder, motorInsurable, pack);
System.out.println(tariffication.getTotalPremiumGross());
} catch(ValidationException e) {
for(Message message : e.getFaultInfo().getReport().getMessage()) {
System.out.println(message.getCode()+" - "+message.getSeverity()+" - "+message.getTarget()+": "+message.getBody());
}
}
}
}
```
Being the output:
Request code: req-50045-1400571612142
Premium Gross Amount: 439.31
Premium Net Amount: 313.85
###Example 3
In this example, taken from OneView *(see image above)*, we tariffy package Speed 2 and get an invalid result. Notice that the input is missing some required informations about covers.
*Input*
```
0800012015-11-24000201012940356032IBZ8972101982-08-221500 011genderCode>
953
```
*Output*
```
ns2:ServerΠαρουσιάστηκε σφάλμα κατά την τιμολόγηση.error1/pack/cover[@code="281000000"]
Η κάλυψη με κωδικό: 281000000 είναι υποχρεωτικό να δηλωθεί.
false
```
###Example 4
In this example, we tariffy package Speed 3 and get an invalid result, caused by insurable's wrong data.
*Input*
```
0800012015-11-24000201012940356032IBZ8972101500 011genderCode>
953
```
*Output*
```
ns2:ServerΠαρουσιάστηκε σφάλμα κατά την τιμολόγηση.error2/insurable/driver/birthdate
Το πεδίο δεν πρέπει να είναι κενό.
false
```
###Example 5
In this example, we tariffy package Speed 3 and get an invalid result, caused by a missing deductible.
*Input*
```
0030112015-11-2412345678911962-08-22000201015650356031IBZ8972102011-11-201982-08-221500 011002953950
```
*Output*
```
ns2:ServerΠαρουσιάστηκε σφάλμα κατά την τιμολόγηση.error5/pack/cover[@code="166000000"]/param[@type="GGY"]
Στην κάλυψη με κωδικό: 166000000 η παράμετρος τύπου GGY δεν διαθέτει στοιχείο με κωδικό: null.
false
```
##issue
Issues a quotation for the provided insurable and the selected covers. The quotation is valid within a time window, in which is possible to convert it into a policy.
There are 3 different outcomes (excluding failure):
* A green quotation: The quotation is created correctly and it is ready to become a policy. A bill document and a list of pendencies are provided.
* A yellow quotation: The quotation is created but requires the aproval of an underwriter. It will eventually become green or red. Until then it cannot be converted into a policy. Pendencies can still be uploaded in order to speed up the whole process.
* A red quotation: The quotation is created but contains errors. These errors can be solved and the quotation resubmitted. In case of resubmission though, the quotation should include the quotationCode returned by the issue method. This way our system will understand you want to update the quotation and not just created a new one.
Notice that, regarding the covers, only the *missing* information should be sent. This includes the sum insured, the parameters, and rarely the premium of the covers that are not disabled as well as those covers that are optional (even if they contain no extra input information).
In order to know which coverages should be sent to our system you could:
* Use our [listPackagesInfo](#listPackagesInfo) *(prefered)* or the [getPackageInfo](#getPackageInfo) : Check the list of mandatory and optional covers in order to know which ones can be selected. Check the [cover field](#FieldInfo) type values to know which of them (if any) should be sent with their cover.
* Use OneView as a reference: Check the list of mandatory and optional covers in order to know which ones can be selected. Check the inputs that are capable of taking values from the user (they are not disabled), those are the ones that should be sent along with their cover.
Check the examples to get a better idea of how to use the service.
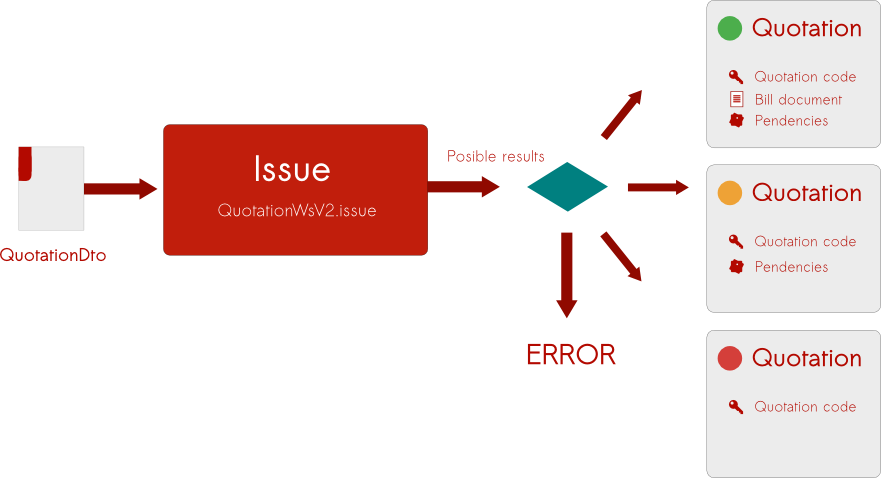
### Input
Field | Type | Value | Required/Optional | Description | Example
----- | ---- | ----- | ----------------- | ----------- | -------
quotation | Quotation | MotorQuotation | Required | The quotation to be issued | *(see below)*
Notice that *Quotation* is an abstract type. **What you should pass as a parameter is the subtype MotorQuotation**. Eventually we may add other types of quotations for the rest of the business areas.
####Quotation
Field | Type | Value | Required/Optional | Description | Example
----- | ---- | ----- | ----------------- | ----------- | ------------ |
distChannelCode | String | Numerical code | Required | The 5 digit code representing the distribution channel | 08000
policyHolder | Person | Person | Required | The policy holder info |*(see example)*
insuranceStartDate | Date | Date | Required | Insurance's start date |2015-10-05
duration | Integer | 1 if annual 2 if semiannual 4 if terminally | Required | The quotation's duration | 1
coverageList | Pack | Pack | Required | The desirable product with coverages |*(see example)*
endorsementCode | String | String | Required | The policy's endorsement policy | 000000000
oldPolicyCode | String | String | Required | The policy's code in case of reneal | empty
insurable | Insurable | MotorInsurable for now | Required | The insurable object |*(see example)*
####MotorInsurable
Field | Type | Value | Required/Optional | Description | Example
--------------------| ---------- | -----------| ------------------- | ---------------------------- | -------
motorUseCode | String | listMotorUses(): code | Required | The motor use code | 000 for E.I.X
motorMakeCode | String | listMotorMakes(): code | Required (Motor packages)| The motor make code | 00007 for CITROEN
eurotaxCode | String | String | Required (Speed packages)| The eurotax code | 46932
plateNo | String | String | Required | The motor's plate no | IBY1234
manufacturerYear | Integer | String | Required | The motor's manufacturer year| 2010
marketValue | BigDecimal | BigDecimal | Required | Motor's market value | 15000
noOfClaims | Integer | Integer | Required | Number of claims | 0
protectionMeasures | Set[String]| listProtectionMeasures(): code | Optional | Protection measures of the vehicle | 001
purchasedDate | Date | Date | Optional | Motor's puschase date | 2010-01-01
licenseDate | Date | Date | Required(Speed packages or in case there is driver) | Driver's lisence date | 2005-01-01
taxHp | Integer | Integer | Required (Speed packages)| Motor's tax hp | 10
cc | Integer | Integer | Required (Motor packages) | Motor's cc | 650
provinceCode | String | listProvinceCodes(): code | Required (Motor packages)| The province code | *(see example)*
driver | Person | String | Optional if policy holder is different from main driver or in Motor packages| Driver info | *(see example)*
####Person
Field | Type | Value | Required/Optional | Description | Example
----- | ---- | ----- | ----------------- | ----------- | -------
gender | int | listGenders():code | Required | The gender of the person | 1
surname | String(1-30 chars) | String | * **Required** *: If the gender is male or female * **Not allowed** *: If the gender is company | The surname of the person | *(see example)*
firstname | String(1-15 chars) | String | * **Required** *: If the gender is male or female * **Not allowed** *: If the gender is company | The name of the person | *(see example)*
fathername | String(1-15 chars) | String | Optional | Father's name | *(see example)*
employmentType | String | listEmployments():code | Optional | The code of the person's employment type | 220
licenseDate | Date | Date | Required | The license date of the person | 20/01/1999
birthDate | Date | Date | * **Required** *: If the gender is male or female * **Optional** *: If the gender is company | The birthdate of the person | 01/01/1987
maritalStatus | int | listMaritalStatuses():code | * **Required** *: If the gender is male or female * **Not allowed** * | The marital status of the person | 3
afm | String(1-9 chars) | Numerical | Required | The Tax Registration Number of the person | 123456789
personId | String | String | Optional | The person's ID number | AI12345
iban1-iban7 | String(1-34 chars) | String | Optional | The digits of the IBAN | 1111
streetName | String(1-30 chars) | String | Required | The street name of the person's residence | *(see example)*
streetNo | String(1-10 chars) | String | Optional | The street number of the person's residence | *(see example)*
postCode | String(1-15 chars) | Numerical | Required | The postal code of the person's residence | *(see example)*
city | String(1-30 chars) | String | Required | The city of the person's residence | *(see example)*
phoneNo | String(1-15 chars) | Numerical | Optional | The phone number (home or mobile) of the person | *(see example)*
fax | String(1-15 chars) | Numerical | Optional | The fax number of the person | *(see example)*
email | String(1-60 chars) | String | Optional | The email of the person | *(see example)*
nameNo | String | Numerical | Optional | The nameNo of a person, if existing | 13734832
####Pack
check *[tariffy](#tariffy)* method.
### Output
#### Quotation report
Field | Type | Description | Example
----- | ---- | ----------- | -------
quotationCode | String | The quotation's code | 00232425
status | String | The quotation's status (e.g GREEN, YELLOW, RED)| GREEN
Report | Report | The report notifying errors while issuing the quotation | *(see example)*
pendencies | List | The list of pendencies (e.g Driver lisence doc) | *(see example)*
#### Pendency
Field | Type | Description | Example
----- | ---- | ----------- | -------
code | String | String | 100071626
type | String | String | 20000
description | String | String | Δεν έχει σταλεί η Αδεια Κυκλοφορίας του Οχήματος
###Example1
Example with yellow quotation
*Input*
```
953950080002017-04-271356032010180000000IKM958902010-01-0110943276841985-01-01ΑΘΗΝΑxxxxxxx@gmail.com22022006-05-061XXXXXXXXXXXΕΛΛΗΝΙΚΗ21088888888104 45ΠΥΘΕΟΥ2XXXX166 75
```
*Output*
```
01981652YELLOW10176411420000Δεν έχει σταλεί η Αδεια Οδήγησης10176411620010Δεν έχει σταλεί η Αδεια Κυκλοφορίας του Οχήματος10176411820030Λοιπά συννημέναWARNING0/quotation
Η ασφαλισμένη αξία έχει διαφορά μεγαλύτερη του 20% από το EUROTAX
WARNING0/quotation
Ο Συμβ/νος έχει ήδη Ασφ/ριο με ζημιές, άκυρο από την Εταιρία
true
```
###Example2
Example with green quotation
*Input*
```
953950080002017-04-271356032010120000000IKM955002010-01-0111235642961985-01-01ΑΘΗΝΑxxxxxxx@gmail.com22022006-05-061XXXXXXXXXXXΕΛΛΗΝΙΚΗ21088888888104 45ΠΥΘΕΟΥ2XXXX166 75
```
*Output*
```
01981662GREEN10176419420000Δεν έχει σταλεί η Αδεια Οδήγησης10176419620010Δεν έχει σταλεί η Αδεια Κυκλοφορίας του Οχήματος10176419820030Λοιπά συννημέναtrue
```
###Example3
Example with a RED quotation
*Input*
```
953950080002017-04-271356032010120000000IKM955002010-01-0111235642951985-01-01ΑΘΗΝΑxxxxxxx@gmail.com22022006-05-061XXXXXXXXXXXΕΛΛΗΝΙΚΗ21088888888104 45ΠΥΘΕΟΥ2XXXX166 75
```
*Output*
```
01981663REDERROR0/quotation
Το Α.Φ.Μ. του Συμβαλλομένου είναι λάθος
false
```
### Code example (in Java)
```
public static final void main(String[] args) {
Soap_002fV2_002fQuotation service = new Soap_002fV2_002fQuotation();
QuotationWsV2 quotationService = service.getQuotationWsV2BasePort();
Map context = ((BindingProvider)quotationService).getRequestContext();
context.put(BindingProvider.USERNAME_PROPERTY, "ws-000000028ezr9");
context.put(BindingProvider.PASSWORD_PROPERTY, "CP64UpfDVZ-test");
Person policyHolder = new Person();
policyHolder.setBirthDate(date(1972, 4, 12));
policyHolder.setMaritalStatusCode(3);
policyHolder.setGenderCode(1);
policyHolder.setPostalCode("104 45");
policyHolder.setAfm("154452074");
policyHolder.setEmploymentTypeCode("220");
policyHolder.setStreetName("Ermou");
Person driver = new Person();
driver.setBirthDate(date(1982, 8, 22));
driver.setMaritalStatusCode(1);
driver.setGenderCode(1);
driver.setPostalCode("104 45");
driver.setAfm("045319514");
driver.setEmploymentTypeCode("220");
driver.setStreetName("Ramblas");
MotorInsurable motorInsurable = new MotorInsurable();
motorInsurable.setMotorUseCode("000");
motorInsurable.setEurotaxCode("35603");
motorInsurable.setManufacturerYear(2010);
motorInsurable.setMarketValue(BigDecimal.valueOf(15650));
motorInsurable.setPlateNo("IBZ8976");
motorInsurable.setNoOfClaims(2);
motorInsurable.setDriver(driver);
// Fill mandatory Cover for Personal Accident
Cover personalAccident = new Cover();
Value sumInsuredPersonal = new Value();
sumInsuredPersonal.setCodeValue("953");
personalAccident.setCode("280000000");
personalAccident.setSumInsured(sumInsuredPersonal);
// Fill mandatory Cover for Windscreen
Cover windscreen = new Cover();
Value sumInsuredWindscreen = new Value();
sumInsuredWindscreen.setCodeValue("950");
windscreen.setCode("281000000");
windscreen.setSumInsured(sumInsuredWindscreen);
// Fill optional Cover for Motor Assistance
Cover motorAssistance = new Cover();
motorAssistance.setCode("753450171");
Pack pack = new Pack();
pack.setCode("802");
pack.getCover().add(personalAccident);
pack.getCover().add(windscreen);
pack.getCover().add(motorAssistance);
MotorQuotation quotation = new MotorQuotation();
quotation.setDistChannelCode("08000");
quotation.setDuration(1);
quotation.setInsuranceStartDate(date(2015, 11, 23));
quotation.setMotorInsurable(motorInsurable);
quotation.setPack(pack);
quotation.setPolicyHolder(policyHolder);
try {
QuotationCreateReport report = quotationService.issue(quotation);
System.out.println(report.getStatus());
} catch(ValidationException e) {
for(Message message : e.getFaultInfo().getReport().getMessage()) {
System.out.println(message.getCode()+" - "+message.getSeverity()+" - "+message.getTarget()+": "+message.getBody());
}
}
}
```
##listPackagesInfo
Provides information about the selectable packages. This information includes the covers and parameters that should be sent to our system when performing tariffication or issuing policies. Pay notice that packages (801-804) are reffered to E.I.X motoruse only and 003 refers to every other use than E.I.X.
### Output
Field | Type | Value | Description | Example
----- | ---- | ----- | ----------- | -------
packageInfo | List[PackageInfo] | PackageInfo | A List of the available packages | *(see below)*
#### PackageInfo
Field | Type | Value | Description | Example
----- | ---- | ----- | ----------- | -------
code | String | Numerical | The code of the package | 801
description | String | Textual | The description of the package | Speed1
cover | List[CoverInfo] | List[CoverInfo] | The products belonging to the package | *(see example)*
#### ProductInfo
Field | Type | Value | Description | Example
----- | ---- | ----- | ----------- | -------
code | String | Numerical | The code of the product | 001
description | String | Textual | The description of the product | Product 1
mandatory | Boolean | Boolean | Defines if the product is mandatory or optional | false
sumInsured | FieldInfo | FieldInfo | The information about the sum insured | ["automatic", Value[3000], List[]]
premium | FieldInfo | FieldInfo | The information about the premium | ["automatic", Value[3000], List[ Param["001", "MERCEDES"], Param["002", "AUDI"]]]
params | List[ParamInfo] | ParamInfo | The parameters of the product | ["001", "Extra Param1", Value[1000], List[ Param["001", "ALARM"] ]]
#### FieldInfo
Field | Type | Value | Description | Example
----- | ---- | ----- | ----------- | -------
type | String | automatic, typeIn, none, param | The type of the field | automatic
value | Value | Value | The value of the field | Value[3000]
options | list[Param] | Param | Possible options of the field | *(see General considerations)*
About the type element:
The FieldInfo *type* refers to the kind of value that the field holds.
Type | Description
---- | -----------
typeIn | A numerical value, freely chosen. The value can still be negatively validated from our business rules.
param | A code value, to be chosen among a provided list. The list of options is provided with the field.
automatic | A value that is not chosen by the client application but outputted by our system.
none | There is no value assignable, neither from the client, neither from our system.
#### ParamInfo
Field | Type | Value | Description | Example
----- | ---- | ----- | ----------- | -------
type | String | String | Specifies the type of the parameter | 001
label | String | String | The label of the parameter | Extra Param1
value | Value | Value | The value of the parameter | Value[1000]
options | List[Param] | Param | Options of the parameter | *(see General considerations)*
###Example
```
Πακέτο Speed1Α.Ε.-Σωμ.ΒλάβεςtrueautomaticautomaticΑ.Ε.-Υλ.ΖημιέςtrueautomaticautomaticΑ.Ε.στη μεταφ.& σε φυλ.χώρουςtruenoneautomaticΥλ.Ζημιές από ανασφ.όχημαtrueautomaticautomaticΚάλυψη αερόσακωνtrueautomaticautomaticΝομική ΣτήριξηtrueautomaticautomaticΦροντίδα ΑτυχήματοςtruenoneautomaticΡυμούλκ.λόγω ατυχ.truenoneautomaticΠροσωπικό ΑτύχημαtrueparamautomaticΠυρκαγιάtrueautomaticautomaticΑ.Ε.από πυρκαγιάtrueautomaticautomaticΟλική ΚλοπήtrueautomaticautomaticΜερική ΚλοπήtrueautomaticautomaticΕνοικ.αυτοκ.λόγω πυρκ.-ολ.κλ.trueautomaticautomaticΦυσικά ΦαινόμεναtrueautomaticautomaticGGYΑπαλλαγήΘραύση ΚρυστάλλωνtrueparamautomaticΑντικατάσταση κλειδιώνtrueautomaticautomaticΑπώλεια εγγράφωνtrueautomaticautomaticΠροστασία αξίας οχήματοςfalseautomaticautomaticΟδική ΒοήθειαfalseautomaticautomaticΑ.Ε.ΡυμουλκούμενουfalseautomaticautomaticΝέος σε ηλικία οδηγόςfalseautomaticautomaticΝέος σε δίπλωμα οδηγόςfalseautomaticautomaticΠακέτο Speed2Α.Ε.-Σωμ.ΒλάβεςtrueautomaticautomaticΑ.Ε.-Υλ.ΖημιέςtrueautomaticautomaticΑ.Ε.στη μεταφ.& σε φυλ.χώρουςtruenoneautomaticΥλ.Ζημιές από ανασφ.όχημαtrueautomaticautomaticΚάλυψη αερόσακωνtrueautomaticautomaticΝομική ΣτήριξηtrueautomaticautomaticΦροντίδα ΑτυχήματοςtruenoneautomaticΡυμούλκ.λόγω ατυχ.truenoneautomaticΠροσωπικό ΑτύχημαtrueparamautomaticΠυρκαγιάtrueautomaticautomaticΑ.Ε.από πυρκαγιάtrueautomaticautomaticΟλική ΚλοπήtrueautomaticautomaticΜερική ΚλοπήtrueautomaticautomaticΕνοικ.αυτοκ.λόγω πυρκ.-ολ.κλ.trueautomaticautomaticΦυσικά ΦαινόμεναtrueautomaticautomaticGGYΑπαλλαγήΘραύση ΚρυστάλλωνtrueparamautomaticΑντικατάσταση κλειδιώνtrueautomaticautomaticΑπώλεια εγγράφωνtrueautomaticautomaticΠροστασία αξίας οχήματοςfalseautomaticautomaticΟδική ΒοήθειαfalseautomaticautomaticΑ.Ε.ΡυμουλκούμενουfalseautomaticautomaticΝέος σε ηλικία οδηγόςfalseautomaticautomaticΝέος σε δίπλωμα οδηγόςfalseautomaticautomaticΠακέτο Speed3Α.Ε.-Σωμ.ΒλάβεςtrueautomaticautomaticΑ.Ε.-Υλ.ΖημιέςtrueautomaticautomaticΑ.Ε.στη μεταφ.& σε φυλ.χώρουςtruenoneautomaticΥλ.Ζημιές από ανασφ.όχημαtrueautomaticautomaticΚάλυψη αερόσακωνtrueautomaticautomaticΝομική ΣτήριξηtrueautomaticautomaticΦροντίδα ΑτυχήματοςtruenoneautomaticΡυμούλκ.λόγω ατυχ.truenoneautomaticΠροσωπικό ΑτύχημαtrueparamautomaticΠυρκαγιάtrueautomaticautomaticΑ.Ε.από πυρκαγιάtrueautomaticautomaticΟλική ΚλοπήtrueautomaticautomaticΜερική ΚλοπήtrueautomaticautomaticΕνοικ.αυτοκ.λόγω πυρκ.-ολ.κλ.trueautomaticautomaticΦυσικά ΦαινόμεναtrueautomaticautomaticGGYΑπαλλαγήΘραύση ΚρυστάλλωνtrueparamautomaticΑντικατάσταση κλειδιώνtrueautomaticautomaticΑπώλεια εγγράφωνtrueautomaticautomaticΠροστασία αξίας οχήματοςfalseautomaticautomaticΟδική ΒοήθειαfalseautomaticautomaticΑ.Ε.ΡυμουλκούμενουfalseautomaticautomaticΝέος σε ηλικία οδηγόςfalseautomaticautomaticΝέος σε δίπλωμα οδηγόςfalseautomaticautomaticΠακέτο MotorΑ.Ε.-Σωμ.ΒλάβεςtrueautomaticautomaticΑ.Ε.-Υλ.ΖημιέςtrueautomaticautomaticΑ.Ε.στη μεταφ.& σε φυλ.χώρουςtruenoneautomaticΥλ.Ζημιές από ανασφ.όχημαtrueautomaticautomaticΚάλυψη αερόσακωνtrueautomaticautomaticΝομική ΣτήριξηtrueautomaticautomaticΦροντίδα ΑτυχήματοςtruenoneautomaticΡυμούλκ.λόγω ατυχ.truenoneautomaticΠροσωπικό ΑτύχημαtrueparamautomaticΠυρκαγιάtrueautomaticautomaticΑ.Ε.από πυρκαγιάtrueautomaticautomaticΟλική ΚλοπήtrueautomaticautomaticΜερική ΚλοπήtrueautomaticautomaticΕνοικ.αυτοκ.λόγω πυρκ.-ολ.κλ.trueautomaticautomaticΦυσικά ΦαινόμεναtrueautomaticautomaticGGYΑπαλλαγήΘραύση ΚρυστάλλωνtrueparamautomaticΑντικατάσταση κλειδιώνtrueautomaticautomaticΑπώλεια εγγράφωνtrueautomaticautomaticΠροστασία αξίας οχήματοςfalseautomaticautomaticΟδική ΒοήθειαfalseautomaticautomaticΑ.Ε.ΡυμουλκούμενουfalseautomaticautomaticΝέος σε ηλικία οδηγόςfalseautomaticautomaticΝέος σε δίπλωμα οδηγόςfalseautomaticautomatic
```
##getPackageInfo
Provides information about a selected package. This information includes the covers and parameters that should be sent to our system when performing tariffication or issuing policies.
### Input
Field | Type | Value | Required/Optional | Description | Example
----- | ---- | ----- | ----------------- | ----------- | -------
packageCode | String | Numerical | required | The code of the requesting package | 801
### Output
Field | Type | Value | Description | Example
----- | ---- | ----- | ----------- | -------
packageInfo | PackageInfo | PackageInfo | A List of the available packages | *(see listPackageInfo)*
###Example 1
**Valid Example**
*Input*
```
801
```
*Output*
```
Πακέτο Speed1Α.Ε.-Σωμ.ΒλάβεςtrueautomaticautomaticΑ.Ε.-Υλ.ΖημιέςtrueautomaticautomaticΑ.Ε.στη μεταφ.& σε φυλ.χώρουςtruenoneautomaticΥλ.Ζημιές από ανασφ.όχημαtrueautomaticautomaticΚάλυψη αερόσακωνtrueautomaticautomaticΝομική ΣτήριξηtrueautomaticautomaticΦροντίδα ΑτυχήματοςtruenoneautomaticΡυμούλκ.λόγω ατυχ.truenoneautomaticΠροσωπικό ΑτύχημαtrueparamautomaticΠυρκαγιάtrueautomaticautomaticΑ.Ε.από πυρκαγιάtrueautomaticautomaticΟλική ΚλοπήtrueautomaticautomaticΜερική ΚλοπήtrueautomaticautomaticΕνοικ.αυτοκ.λόγω πυρκ.-ολ.κλ.trueautomaticautomaticΦυσικά ΦαινόμεναtrueautomaticautomaticGGYΑπαλλαγήΘραύση ΚρυστάλλωνtrueparamautomaticΑντικατάσταση κλειδιώνtrueautomaticautomaticΑπώλεια εγγράφωνtrueautomaticautomaticΠροστασία αξίας οχήματοςfalseautomaticautomaticΟδική ΒοήθειαfalseautomaticautomaticΑ.Ε.ΡυμουλκούμενουfalseautomaticautomaticΝέος σε ηλικία οδηγόςfalseautomaticautomaticΝέος σε δίπλωμα οδηγόςfalseautomaticautomatic
```
###Example 2
**Example with error**
*Input*
```
1
```
*Output*
```
S:ServerInvalid package code: 1
```
##listOpenPendencies
This method is responsible for listing all open pendencies given the quotation code.
### Input
Field | Type | Value | Required/Optional | Description | Example
----- | ---- | ----- | ----------------- | ----------- | -------
quotationCode | String | Numerical | required | The quotation code | 00915180
### Output
Field | Type | Value | Description | Example
----- | ---- | ----- | ----------- | -------
pendencies | List | The list of pendencies (e.g Driver lisence doc) | *(see example)*
#### Pendency
Field | Type | Description | Example
----- | ---- | ----------- | -------
code | String | String | 100071626
type | String | String | 20000
description | String | String | Δεν έχει σταλεί η Αδεια Κυκλοφορίας του Οχήματος
###Example 1
*Input*
```
00915180
```
*Output*
```
10007159620000Δεν έχει σταλεί η Αδεια Οδήγησης10007159820010Δεν έχει σταλεί η Αδεια Κυκλοφορίας του Οχήματος
```
##uploadPendency
### Input
Field | Type | Value | Required/Optional | Description | Example
----- | ---- | ----- | ----------------- | ----------- | -------
pendencyCode | String | listOpenPendencies | Required | The pendency code| 100071596
quotationCode | String | Numerical | Required | The code of the requesting package | 00915180
title | String | String | Required | The desired title of the uploaded document | adeia_odigisis_xxxx
document | byte[] | byte[] | Required | The byte[] of the document | *(see example)*
###Example 1
*Input*
```
10007159600915180adeia_odigisis_blabla.pdfcid:adeiaodigisis_blabla.pdf
```
*Output*
```
```
##getQuotationDocument
This method is responsible for getting the quotation document in byte[] format
### Input
Field | Type | Value | Required/Optional | Description | Example
----- | ---- | ----- | ----------------- | ----------- | -------
quotationCode | String | Numerical | required | The quotation code | 00915180
### Output
Field | Type | Value | Description | Example
----- | ---- | ----- | ----------- | -------
document | byte[] | byte[] | get quotation document in byte[] format | *(see example)*
###Example 1
*Input*
```
00915180
```
*Output*
```
JVBERi0...oyMDYzNzYNCiUlRU9GDQo=
```
##getQuotationStatus
This method is responsible for getting the status of quotation (GREEN, YELLOW, RED)
### Input
Field | Type | Value | Required/Optional | Description | Example
----- | ---- | ----- | ----------------- | ----------- | -------
quotationCode | String | Numerical | required | The quotation code | 01243410
### Output
Type | Description | Example
----- | ---- | ----- | ----------- | -------
String| get quotation status | *(see example)*
###Example 1
*Input*
```
01243410
```
*Output*
```
GREEN
```